-
Notifications
You must be signed in to change notification settings - Fork 0
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
- Loading branch information
1 parent
6cf0861
commit daf23cb
Showing
16 changed files
with
380 additions
and
115 deletions.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -1,5 +1,8 @@ | ||
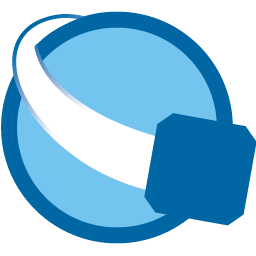 | ||
|
||
### Get Started | ||
### Get Started with Quartus | ||
|
||
> This text will be visible in the builtin package manager | ||
1. Install this Extension | ||
2. Download and Install [Quartus for Linux](https://download.altera.com/akdlm/software/acdsinst/18.1std/625/ib_installers/QuartusLiteSetup-18.1.0.625-linux.run) or for [Quartus for Windows](https://download.altera.com/akdlm/software/acdsinst/18.1std/625/ib_installers/QuartusLiteSetup-18.1.0.625-windows.exe) | ||
3. Go to Settings -> Tools and set the correct installation path | ||
4. Select Quartus as your toolchain in the compile drop-down menu or create a new project selecting quartus |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Loading
Sorry, something went wrong. Reload?
Sorry, we cannot display this file.
Sorry, this file is invalid so it cannot be displayed.
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,119 @@ | ||
using System.Text.RegularExpressions; | ||
using OneWare.Essentials.Extensions; | ||
using OneWare.Essentials.Models; | ||
|
||
namespace OneWare.Quartus.Helper; | ||
|
||
public partial class QsfFile(string[] lines) | ||
{ | ||
[GeneratedRegex(@"set_location_assignment\s*PIN_(\w+)\s+-to\s+(\w+)")] | ||
private static partial Regex LocationAssignmentRegex(); | ||
|
||
[GeneratedRegex(@"set_location_assignment\s")] | ||
private static partial Regex RemoveLocationAssignmentRegex(); | ||
|
||
public List<string> Lines { get; private set; } = lines.ToList(); | ||
|
||
public string? GetGlobalAssignment(string propertyName) | ||
{ | ||
var regex = new Regex(@"set_global_assignment\s*-name\s" + propertyName + @"\s(.+)"); | ||
foreach (var line in Lines) | ||
{ | ||
var match = regex.Match(line); | ||
if (match is { Success: true, Groups.Count: > 1 }) | ||
{ | ||
var value = match.Groups[1].Value; | ||
if(value.Length > 0 && value[0] == '"' && value[^1] == '"') return value[1..^1]; | ||
return value; | ||
} | ||
} | ||
return null; | ||
} | ||
|
||
public void SetGlobalAssignment(string name, string value) | ||
{ | ||
var regex = new Regex(@"set_global_assignment\s*-name\s*" + name); | ||
var line= Lines.FindIndex(x => regex.IsMatch(x)); | ||
var newAssignment = $"set_global_assignment -name {name} \"{value}\""; | ||
|
||
if(line != -1) | ||
{ | ||
Lines[line] = newAssignment; | ||
} | ||
else | ||
{ | ||
Lines.Add(newAssignment); | ||
} | ||
} | ||
|
||
public void RemoveGlobalAssignment(string name) | ||
{ | ||
var regex = new Regex(@"set_global_assignment\s*-name\s*" + name); | ||
Lines = Lines.Where(x => !regex.IsMatch(x)).ToList(); | ||
} | ||
|
||
public IEnumerable<(string,string)> GetLocationAssignments() | ||
{ | ||
foreach (var line in lines) | ||
{ | ||
var match = LocationAssignmentRegex().Match(line); | ||
if (!match.Success) continue; | ||
|
||
var pin = match.Groups[1].Value; | ||
var node = match.Groups[2].Value; | ||
|
||
yield return (pin, node); | ||
} | ||
} | ||
|
||
public void AddLocationAssignment(string pin, string node) | ||
{ | ||
Lines.Add($"set_location_assignment PIN_{pin} -to {node}"); | ||
} | ||
|
||
public void RemoveLocationAssignments() | ||
{ | ||
var regex = RemoveLocationAssignmentRegex(); | ||
Lines = Lines.Where(x => !regex.IsMatch(x)).ToList(); | ||
} | ||
|
||
public void AddFile(IProjectFile file) | ||
{ | ||
switch (file.Extension) | ||
{ | ||
case ".vhd" or ".vhdl": | ||
Lines.Add($"set_global_assignment -name VHDL_FILE {file.RelativePath.ToLinuxPath()}"); | ||
break; | ||
case ".v": | ||
Lines.Add($"set_global_assignment -name VERILOG_FILE {file.RelativePath.ToLinuxPath()}"); | ||
break; | ||
case ".sv": | ||
Lines.Add($"set_global_assignment -name SYSTEMVERILOG_FILE {file.RelativePath.ToLinuxPath()}"); | ||
break; | ||
case ".qip": | ||
Lines.Add($"set_global_assignment -name QIP_FILE {file.RelativePath.ToLinuxPath()}"); | ||
break; | ||
case ".qsys": | ||
Lines.Add($"set_global_assignment -name QSYS_FILE {file.RelativePath.ToLinuxPath()}"); | ||
break; | ||
case ".bdf": | ||
Lines.Add($"set_global_assignment -name BDF_FILE {file.RelativePath.ToLinuxPath()}"); | ||
break; | ||
case ".ahdl": | ||
Lines.Add($"set_global_assignment -name AHDL_FILE {file.RelativePath.ToLinuxPath()}"); | ||
break; | ||
case ".smf": | ||
Lines.Add($"set_global_assignment -name SMF_FILE {file.RelativePath.ToLinuxPath()}"); | ||
break; | ||
case ".tcl": | ||
Lines.Add($"set_global_assignment -name TCL_SCRIPT_FILE {file.RelativePath.ToLinuxPath()}"); | ||
break; | ||
case ".hex": | ||
Lines.Add($"set_global_assignment -name HEX_FILE {file.RelativePath.ToLinuxPath()}"); | ||
break; | ||
case ".mif": | ||
Lines.Add($"set_global_assignment -name MIF_FILE {file.RelativePath.ToLinuxPath()}"); | ||
break; | ||
} | ||
} | ||
} |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,24 @@ | ||
using System.Text.RegularExpressions; | ||
using OneWare.UniversalFpgaProjectSystem.Models; | ||
|
||
namespace OneWare.Quartus.Helper; | ||
|
||
public static partial class QsfHelper | ||
{ | ||
public static string GetQsfPath(UniversalFpgaProjectRoot project) | ||
{ | ||
return Path.Combine(project.RootFolderPath, Path.GetFileNameWithoutExtension(project.TopEntity?.FullPath ?? throw new Exception("TopEntity not set!")) + ".qsf"); | ||
} | ||
|
||
public static QsfFile ReadQsf(string path) | ||
{ | ||
var qsf = File.Exists(path) ? File.ReadAllText(path) : string.Empty; | ||
|
||
return new QsfFile(qsf.Split('\n', StringSplitOptions.RemoveEmptyEntries | StringSplitOptions.TrimEntries)); | ||
} | ||
|
||
public static void WriteQsf(string path, QsfFile file) | ||
{ | ||
File.WriteAllLines(path, file.Lines); | ||
} | ||
} |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Oops, something went wrong.