-
Notifications
You must be signed in to change notification settings - Fork 12
New issue
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement. We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
Typescript support #29
Comments
For anyone who needs it. Here's my best attempt so far for the workaround: Add to any declare module '@twilio/twilio-verify-for-react-native' {
export interface Metadata {
nextPageToken?: string
page: number
pageSize: number
previousPageToken?: string
}
// eslint-disable-next-line @typescript-eslint/no-explicit-any
export type JSONObject = { [key: string]: any }
export interface Challenge {
challengeDetails: ChallengeDetails
createdAt: Date
expirationDate: Date
factorSid: string
hiddenDetails: JSONObject
sid: string
status: ChallengeStatus
updatedAt: Date
}
export interface ChallengeList {
challenges: Challenge[]
metadata: Metadata
}
export enum ChallengeListOrder {
Asc = 'asc',
Desc = 'desc',
}
export enum ChallengeStatus {
Pending = 'pending',
Approved = 'approved',
Denied = 'denied',
Expired = 'expired',
}
export interface ChallengeDetails {
date?: Date
fields: Detail[]
message: string
}
export interface Detail {
label: string
value: string
}
export interface Factor {
accountSid: string
createdAt: Date
friendlyName: string
identity: string
serviceSid: string
sid: string
status: FactorStatus
type: FactorType
}
enum FactorType {
Push = 'push',
}
export enum FactorStatus {
Verified = 'verified',
Unverified = 'unverified',
}
/*
Create factor method
*/
// eslint-disable-next-line @typescript-eslint/no-extraneous-class
declare class PushFactorPayload {
/**
* Used as a parameter for the `createFactor()` method.
*
* @param factorName - Any friendly name
* @param verifyServiceSid - The service SID (e.g. VA00f7e870e0ebc27c85ca8ab75e2c770d, from Twilio Verify's dashboard)
* @param identity - A unique identifier (usually a user's UUID)
* @param accessToken - Access token by authentication with Twilio
* @param pushToken - Your device's FCM or APNS token
* @returns A payload object
*
*/
constructor(
factorName: string,
verifyServiceSid: string,
identity: string,
accessToken: string,
pushToken: string,
)
}
/**
* Used to create a factor for your device.
*
* @param payload - Created with the `PushFactorPayload` class
* @returns A Factor
*
*/
export function createFactor(payload: PushFactorPayload): Promise<Factor>
/*
Verify factor method
*/
// eslint-disable-next-line @typescript-eslint/no-extraneous-class
declare class VerifyPushFactorPayload {
/**
* Used as a parameter for the `createFactor()` method.
*
* @param factorSid - The factor SID
* @returns A payload object
*
*/
constructor(factorSid: string)
}
/**
* Used to verify a factor for your device, that you previously created with the `createFactor()` method, or a factor received by the backend.
*
* @param payload - Created with the `VerifyPushFactorPayload` class
* @returns A Factor
*
*/
export function verifyFactor(
payload: VerifyPushFactorPayload,
): Promise<Factor>
/*
Update challenge method
*/
// eslint-disable-next-line @typescript-eslint/no-extraneous-class
declare class UpdatePushChallengePayload {
/**
* Used as a parameter for the `updateChallenge()` method.
*
* @param factorSid - The factor SID
* @param challengeSid - The challenge SID
* @param newStatus - The new status of the challenge to be updated
* @returns A payload object
*
*/
constructor(
factorSid: string,
challengeSid: string,
newStatus: ChallengeStatus,
)
}
/**
* Used to update a challenge
*
* @param payload - Created with the `UpdatePushChallengePayload` class
* @returns A Challenge
*
*/
export function updateChallenge(
payload: UpdatePushChallengePayload,
): Promise<Challenge>
/*
Get all challenges method
*/
// eslint-disable-next-line @typescript-eslint/no-extraneous-class
declare class ChallengeListPayload {
/**
* Used as a parameter for the `updateChallenge()` method.
*
* @param factorSid - The factor SID
* @param limit - The max number of challenges to return
* @param status - The status of the challenges to return
* @param order - The order of the challenges to return
* @returns A payload object
*
*/
constructor(
public factorSid: string,
public pageSize: number,
public status?: ChallengeStatus,
public order: ChallengeListOrder = ChallengeListOrder.Asc,
public pageToken?: string,
) {}
}
/**
* Used to update a challenge
*
* @param payload - Created with the `ChallengeListPayload` class
* @returns A ChallengeList
*
*/
export function getAllChallenges(
payload: ChallengeListPayload,
): Promise<ChallengeList>
} |
I created Twillio.d.ts and updated @kg-currenxie types:
|
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
Would be nice to get a type definition for all the methods :)
Not sure why it can't load tho.. The node module includes these files:
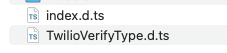
in
./node_modules/@twilio/twilio-verify-for-react-native/lib/typescript
The text was updated successfully, but these errors were encountered: