-
+
+
-
{children}
+
{children}
diff --git a/src/app/dashboard/page.tsx b/src/app/dashboard/page.tsx
index 4a96aef..7d77c27 100644
--- a/src/app/dashboard/page.tsx
+++ b/src/app/dashboard/page.tsx
@@ -1,5 +1,5 @@
"use client";
-
+import type { RootState } from "../store";
import { FileListContext } from "@/app/_context/FilesListContext";
import { api } from "../../../convex/_generated/api";
import { LoginLink, useKindeBrowserClient } from "@kinde-oss/kinde-auth-nextjs";
@@ -11,7 +11,8 @@ import { Input } from "@/components/ui/input";
import ThemeTogglebutton from "@/components/ui/ThemeToggle";
import { Search, Send } from "lucide-react";
import Image from "next/image";
-
+import { setOpen, setClose, toggleClose } from "../Redux/Menu/menuSlice";
+import { useSelector, useDispatch } from "react-redux";
export interface FILE {
archive: boolean;
createdBt: string;
@@ -28,7 +29,8 @@ function Dashboard() {
const { user }: any = useKindeBrowserClient();
const { isAuthenticated } = useKindeBrowserClient();
const createUser = useMutation(api.user.createUser);
-
+ const count = useSelector((state: RootState) => state.counter.value);
+ const dispatch = useDispatch();
const checkUser = async () => {
const result = await convex.query(api.user.getUser, { email: user?.email });
if (!result?.length) {
@@ -55,8 +57,27 @@ function Dashboard() {
};
return isAuthenticated ? (
-
-
+
+
+ {!count && (
+
+ )}
) {
return (
-
-
-
-
- {children}
-
-
-
-
-
+
+
+
+
+
+ {children}
+
+
+
+
+
+
);
}
diff --git a/src/app/store.tsx b/src/app/store.tsx
new file mode 100644
index 0000000..67eb0d0
--- /dev/null
+++ b/src/app/store.tsx
@@ -0,0 +1,12 @@
+import { configureStore } from "@reduxjs/toolkit";
+import counterReducer from "./Redux/Menu/menuSlice";
+export const store = configureStore({
+ reducer: {
+ counter: counterReducer,
+ },
+});
+
+// Infer the `RootState` and `AppDispatch` types from the store itself
+export type RootState = ReturnType
;
+// Inferred type: {posts: PostsState, comments: CommentsState, users: UsersState}
+export type AppDispatch = typeof store.dispatch;
diff --git a/src/components/ui/sheet.tsx b/src/components/ui/sheet.tsx
new file mode 100644
index 0000000..88a3feb
--- /dev/null
+++ b/src/components/ui/sheet.tsx
@@ -0,0 +1,140 @@
+"use client";
+
+import * as React from "react";
+import * as SheetPrimitive from "@radix-ui/react-dialog";
+import { cva, type VariantProps } from "class-variance-authority";
+import { X } from "lucide-react";
+
+import { cn } from "@/lib/utils";
+
+const Sheet = SheetPrimitive.Root;
+
+const SheetTrigger = SheetPrimitive.Trigger;
+
+const SheetClose = SheetPrimitive.Close;
+
+const SheetPortal = SheetPrimitive.Portal;
+
+const SheetOverlay = React.forwardRef<
+ React.ElementRef,
+ React.ComponentPropsWithoutRef
+>(({ className, ...props }, ref) => (
+
+));
+SheetOverlay.displayName = SheetPrimitive.Overlay.displayName;
+
+const sheetVariants = cva(
+ "fixed z-50 gap-4 bg-background p-6 shadow-lg transition ease-in-out data-[state=open]:animate-in data-[state=closed]:animate-out data-[state=closed]:duration-300 data-[state=open]:duration-500",
+ {
+ variants: {
+ side: {
+ top: "inset-x-0 top-0 border-b data-[state=closed]:slide-out-to-top data-[state=open]:slide-in-from-top",
+ bottom:
+ "inset-x-0 bottom-0 border-t data-[state=closed]:slide-out-to-bottom data-[state=open]:slide-in-from-bottom",
+ left: "inset-y-0 left-0 h-full w-3/4 border-r data-[state=closed]:slide-out-to-left data-[state=open]:slide-in-from-left sm:max-w-sm",
+ right:
+ "inset-y-0 right-0 h-full w-3/4 border-l data-[state=closed]:slide-out-to-right data-[state=open]:slide-in-from-right sm:max-w-sm",
+ },
+ },
+ defaultVariants: {
+ side: "right",
+ },
+ }
+);
+
+interface SheetContentProps
+ extends React.ComponentPropsWithoutRef,
+ VariantProps {}
+
+const SheetContent = React.forwardRef<
+ React.ElementRef,
+ SheetContentProps
+>(({ side = "left", className, children, ...props }, ref) => (
+
+
+
+ {children}
+
+
+ Close
+
+
+
+));
+SheetContent.displayName = SheetPrimitive.Content.displayName;
+
+const SheetHeader = ({
+ className,
+ ...props
+}: React.HTMLAttributes) => (
+
+);
+SheetHeader.displayName = "SheetHeader";
+
+const SheetFooter = ({
+ className,
+ ...props
+}: React.HTMLAttributes) => (
+
+);
+SheetFooter.displayName = "SheetFooter";
+
+const SheetTitle = React.forwardRef<
+ React.ElementRef,
+ React.ComponentPropsWithoutRef
+>(({ className, ...props }, ref) => (
+
+));
+SheetTitle.displayName = SheetPrimitive.Title.displayName;
+
+const SheetDescription = React.forwardRef<
+ React.ElementRef,
+ React.ComponentPropsWithoutRef
+>(({ className, ...props }, ref) => (
+
+));
+SheetDescription.displayName = SheetPrimitive.Description.displayName;
+
+export {
+ Sheet,
+ SheetPortal,
+ SheetOverlay,
+ SheetTrigger,
+ SheetClose,
+ SheetContent,
+ SheetHeader,
+ SheetFooter,
+ SheetTitle,
+ SheetDescription,
+};
From e41ad2d6bcaf244b97185dd343bab48f13e6b116 Mon Sep 17 00:00:00 2001
From: Sarthak Mishra <124512392+sarthakmishra459@users.noreply.github.com>
Date: Mon, 13 May 2024 21:20:16 +0530
Subject: [PATCH 2/2] Merge branch 'main' of
https://github.com/sarthakmishra459/BloxAI
---
README.md | 5 +++++
src/app/contributors/ContributorsData.ts | 16 +++++-----------
2 files changed, 10 insertions(+), 11 deletions(-)
diff --git a/README.md b/README.md
index 5e320c2..e1af0b5 100644
--- a/README.md
+++ b/README.md
@@ -45,8 +45,13 @@ Visit the site here - [Blox AI](https://blox-ai.vercel.app/)
## Contributors
+Interested contributors and issue raisers are also requested to join [WhatsApp Group](https://chat.whatsapp.com/E5oRd1VG1Ov4HoNPq4QcRU). For more discussions and faster PR merging
+
To ensure transparency and recognition for your contributions, we've established a straightforward process. When resolving issues or submitting PRs, please remember to fill out your details in `src/app/contributors/ContributorsData.ts`. This file serves as a repository of the individuals who are actively involved in improving our platform.
+Add your Name, Image URL and Github link in this file as an object ->
+[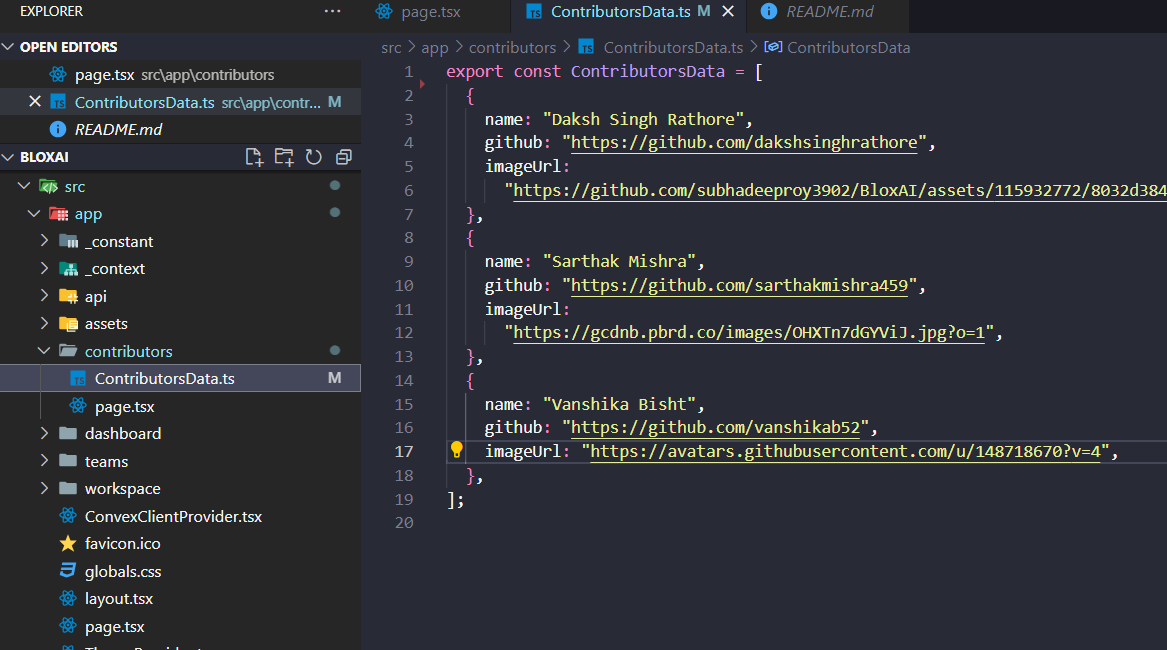](https://postimg.cc/fk0rPV3H)
+
However, it's important to adhere to strict regulations to maintain the integrity of our contributor records:
1. Only Add Your Details: You are allowed to add your own details to the ContributorsData.ts file. This helps us accurately attribute contributions to the right individuals.
diff --git a/src/app/contributors/ContributorsData.ts b/src/app/contributors/ContributorsData.ts
index 0dbfce2..10b99d9 100644
--- a/src/app/contributors/ContributorsData.ts
+++ b/src/app/contributors/ContributorsData.ts
@@ -1,15 +1,4 @@
export const ContributorsData = [
- {
- name: "Anish Biswas",
- github: "https://git.new/Anish",
- imageUrl: "https://blastro.netlify.app/assets/anish.webp",
- },
- {
- name: "Subhadeep Roy",
- github: "https://git.new/subha",
- imageUrl:
- "https://media.licdn.com/dms/image/D4D03AQFalsSBySqQCA/profile-displayphoto-shrink_400_400/0/1680632903861?e=1721260800&v=beta&t=EYuh7f1xzFJv4JerrXg9ho_tTaczzkrDik7KuTOMbTE",
- },
{
name: "Daksh Singh Rathore",
github: "https://github.com/dakshsinghrathore",
@@ -22,4 +11,9 @@ export const ContributorsData = [
imageUrl:
"https://gcdnb.pbrd.co/images/OHXTn7dGYViJ.jpg?o=1",
},
+ {
+ name: "Vanshika Bisht",
+ github: "https://github.com/vanshikab52",
+ imageUrl: "https://avatars.githubusercontent.com/u/148718670?v=4",
+ },
];