diff --git a/examples/README.md b/examples/README.md
new file mode 100644
index 0000000..1c53abb
--- /dev/null
+++ b/examples/README.md
@@ -0,0 +1,11 @@
+# _Examples_
+
+This is a collection of _basic_ examples
+collated from various sources including
+https://elm-lang.org/examples
+to give you a feel for Elm code.
+
+If you get stuck, please open an issue with a question:
+https://github.com/dwyl/learn-elm/issues
+
+We are happy to help _anyone_ learning Elm to make progress! 😊
diff --git a/tutorials/elm-ui/.gitignore b/tutorials/elm-ui/.gitignore
new file mode 100644
index 0000000..dcaf716
--- /dev/null
+++ b/tutorials/elm-ui/.gitignore
@@ -0,0 +1 @@
+index.html
diff --git a/tutorials/elm-ui/README.md b/tutorials/elm-ui/README.md
new file mode 100644
index 0000000..12a9cb0
--- /dev/null
+++ b/tutorials/elm-ui/README.md
@@ -0,0 +1,717 @@
+# `elm-ui` a Language for _Reliable_ Layout and Interface
+
+_This_ tutorial aims to take a complete beginner -
+who has never seen any `elm-ui` -
+to a basic understanding in 10 minutes.
+
+
+
+## _Why?_ 🤷 ... 😢 🌧 `|>` 😍🌈
+
+Few people _love_ writing CSS.
+Most people just want to build their App
+_without_ the headache of knowing how to position things.
+**`elm-ui`** lets you build beautiful,
+fast and responsive UIs in `elm`,
+without writing _any_ CSS!
+But `elm-ui` goes _way_ beyond _just_ a design system.
+Since it's built using pure `elm` functions,
+it gives you ***compile-time guarantees*** that your layout/styles
+are valid. So not only is it easier/faster to build the UI,
+it makes **extending and _maintaining_** your App ***effortless***!
+
+> @dwyl we _love_ the idea of having semantic, functional and responsive UIs
+with no side effects.
+**Functional CSS** libraries allow anyone on a team
+to change _one_ style on a single element
+without affecting any others.
+For the past few years we have been using the Tachyons library
+see:
+[github.com/dwyl/**learn-tachyons**](https://github.com/dwyl/learn-tachyons)
+and it's been a breath of fresh air.
+Using Tachyons in dozens of projects
+has been _good_ in small teams (_0-12 people_)
+and we have had _far_ fewer UI bugs than before adopting Tachyons.
+_However_ we have found that even with a functional CSS library
+(_that greatly reduces the possibility of cascading side effects_),
+we still see redundant and unnecessary styles
+and _occasionally_ visual bugs are introduced that go undetected.
+No CSS library or pre-processor we know of offers compile-time guarantees
+that the layout/styles applied to a given element are _correct_.
+That's where `elm-ui` comes in and changes the game!
+
+
+
+## _What?_ 💭
+
+`elm-ui` is a design system
+for building semantic UI
+with compile-time guarantees.
+
+
+Matthew Griffith described it eloquently in his Elm Conf talk
+"Building a Toolkit for Design":
+
+[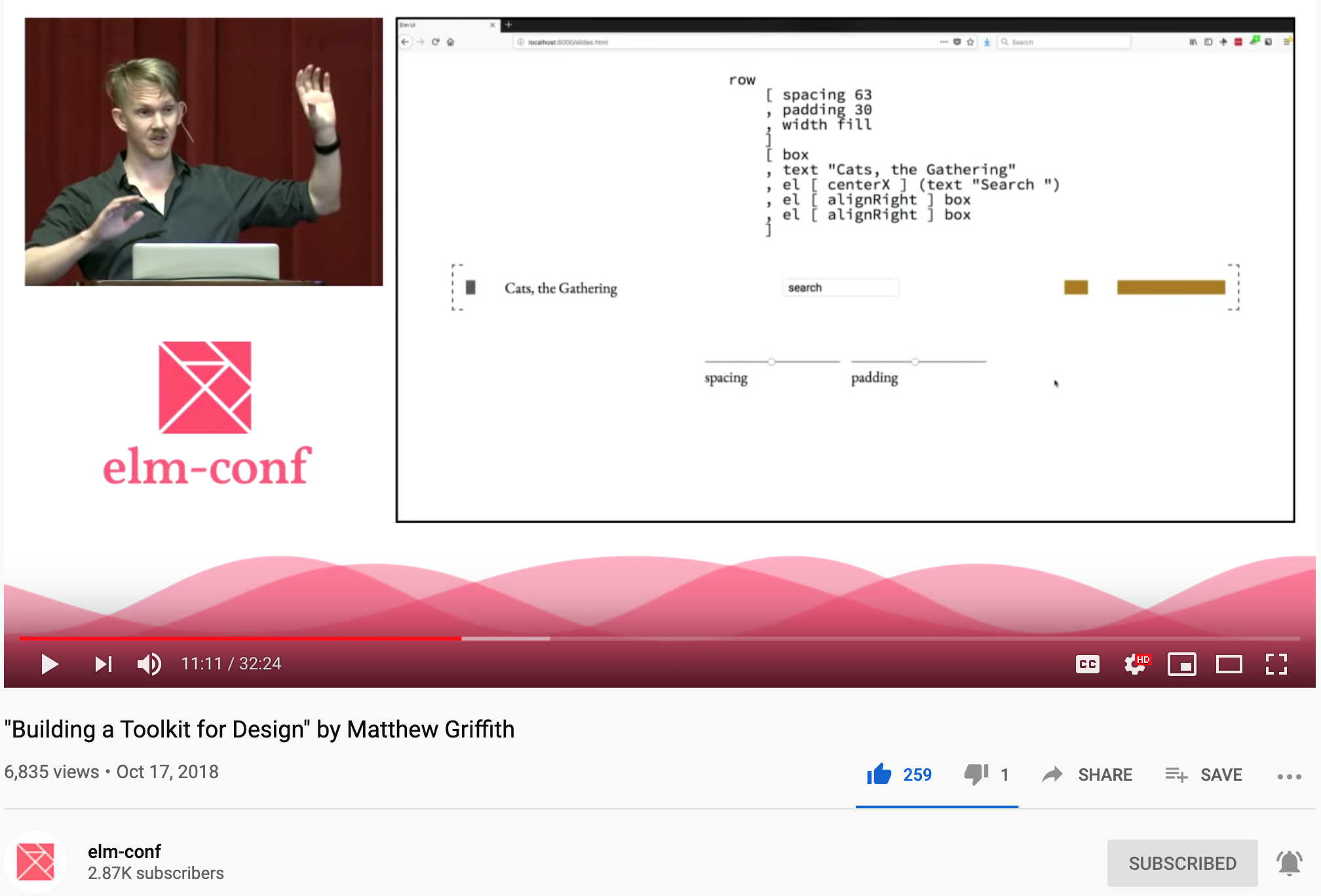](https://youtu.be/Ie-gqwSHQr0)
+https://youtu.be/Ie-gqwSHQr0
+
+> "_It's all right there.
+There's no other place you have to go to modify this_."
+
+
+`elm-ui` is to `HTML` and `CSS`
+what `elm` is to `JavaScript`,
+a way of making designing web applications fun again!
+`elm-ui` offers compile-time guarantees
+that your layout and styles work as expected.
+`elm-ui` will provide you with _helpful_ compiler errors/warnings
+if you attempt to make a _breaking_ change to UI!
+
+
+### Example Compile Time Warnings
+
+In this case I have attempted to apply a `Float`
+of `2.5` to the `Border.rounded` (`border-radius`) property:
+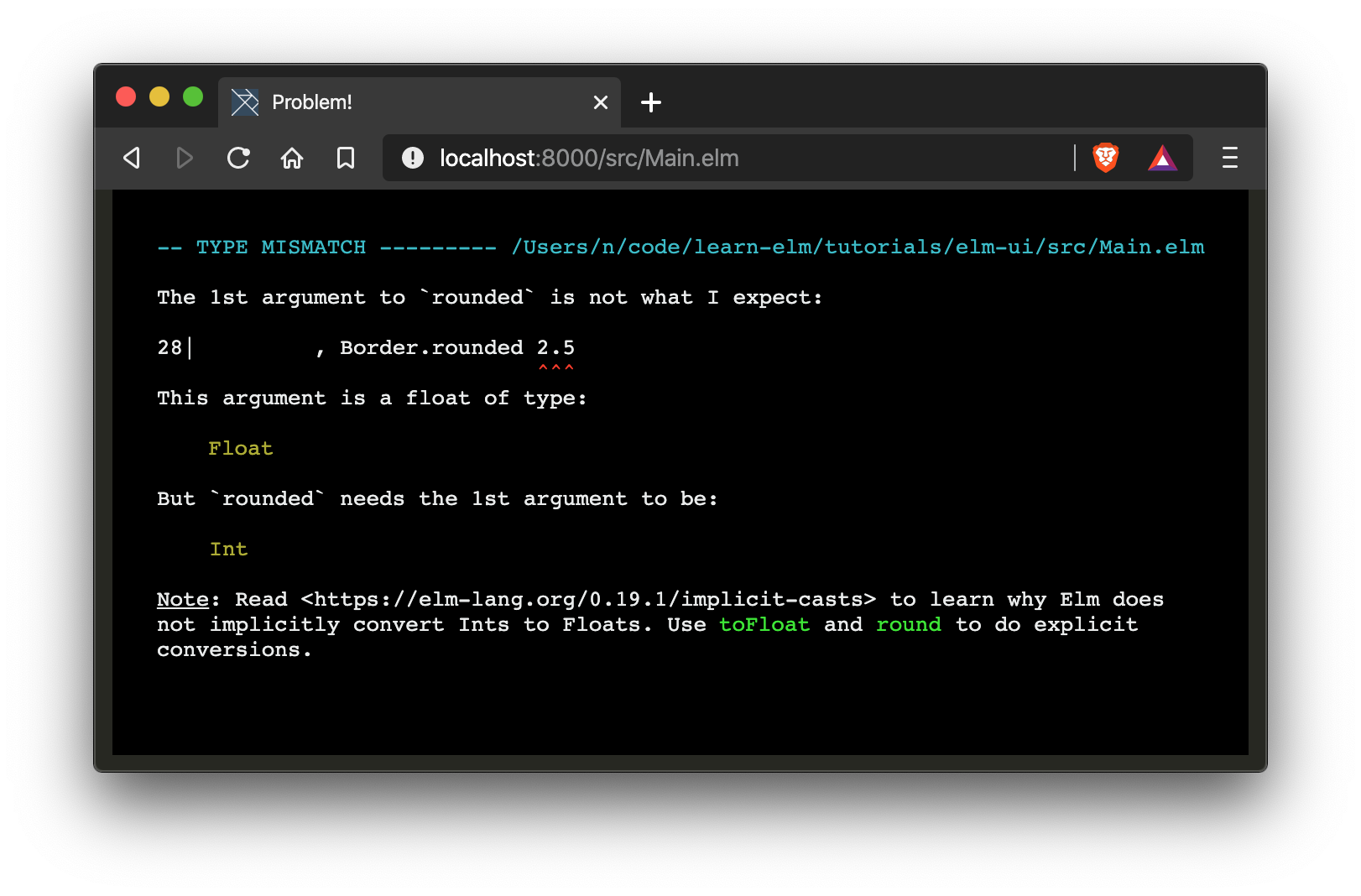
+
+The same thing goes if we attempt to pass a `String`
+to `Border.rounded` e.g: `"2px"`
+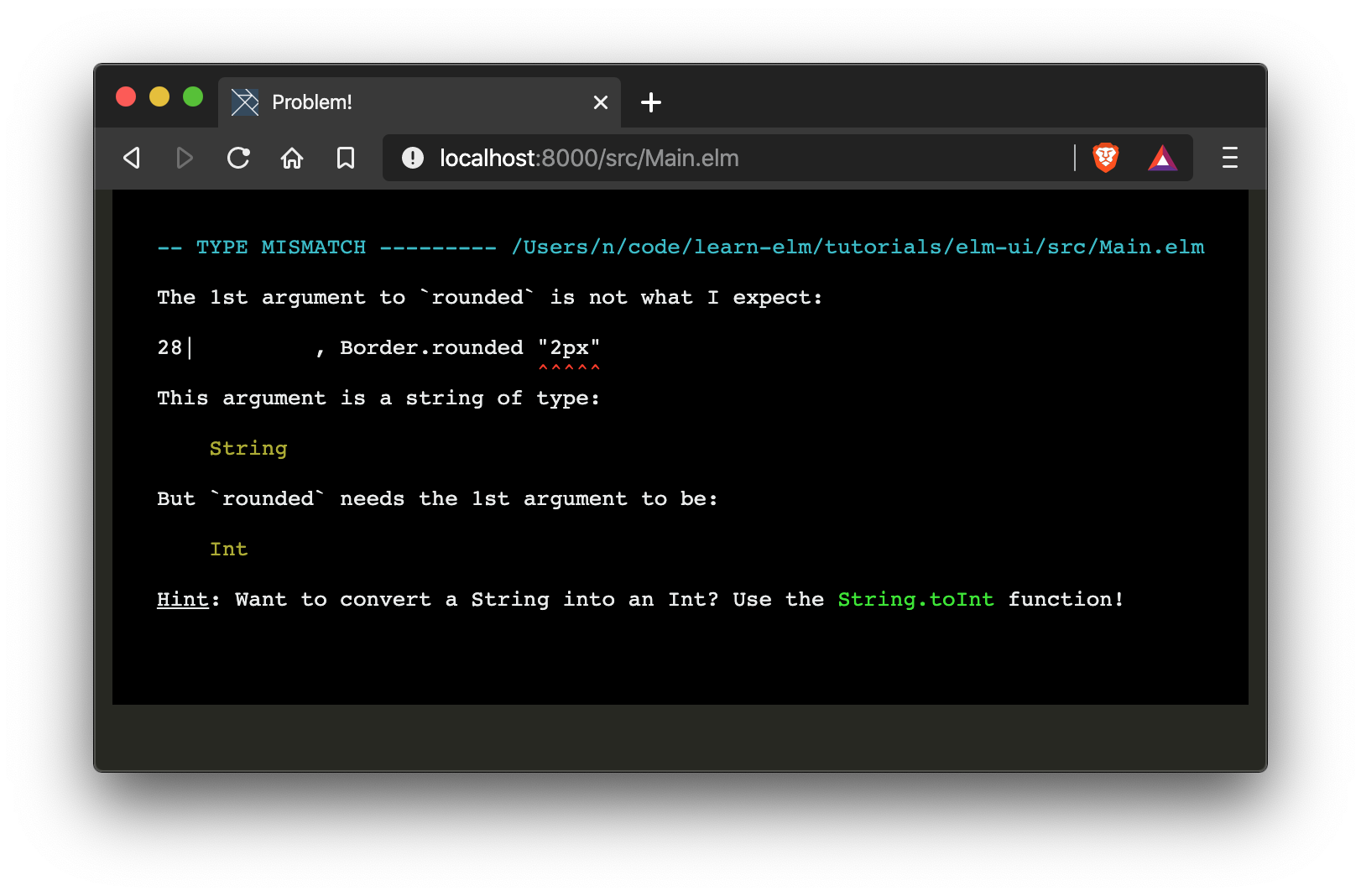
+We get a type mismatch and know _exactly_ what needs to be fixed
+for our view to compile.
+
+If you make a typo in your layout/style it won't compile:
+
+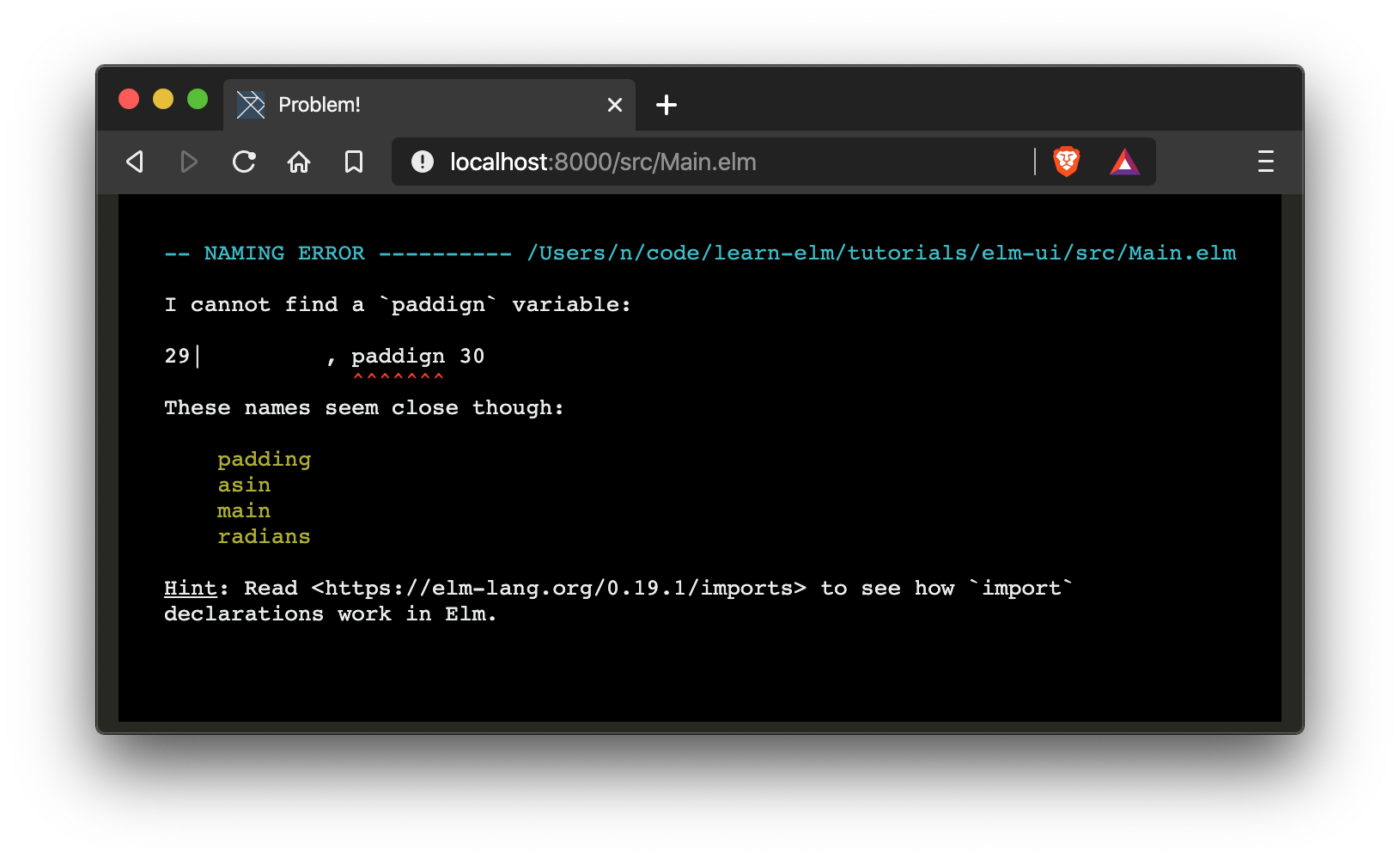
+
+Hopefully that gives you a _taste_
+for the compiler warnings provided by `elm-ui`.
+The best way to experience this further
+is to fire up your text editor and start coding!
+
+
+## _How?_ 📝
+
+In a new directory, initialise your `elm` workflow with the following command:
+```sh
+elm init
+```
+
+That will prompt you to create an `elm.json` file similar to this one:
+[`elm.json`](https://github.com/dwyl/learn-elm/blob/master/tutorials/elm-ui/elm.json)
+
+Next we will add the dependency for `mdgriffith/elm-ui`:
+```sh
+elm install mdgriffith/elm-ui
+```
+> **Note**: that command will only add an entry
+in the `elm.json` `"dependencies"` section.
+`elm install` does not actually _install_ anything
+until you attempt to compile the app.
+
+
+Now create a directory called `src`
+and a new file in the directory `src/Main.elm`.
+Next _type_ (_or copy-paste_) the following code
+into the `src/Main.elm` file:
+
+```elm
+module Main exposing (main)
+
+import Element exposing (Element, alignRight, centerX, centerY, el, fill, padding, rgb255, row, spacing, text, width)
+import Element.Background as Background
+import Element.Border as Border
+import Element.Font as Font
+
+
+main =
+ Element.layout []
+ rowOfStuff
+
+
+rowOfStuff : Element msg
+rowOfStuff =
+ row [ width fill, centerY, spacing 30 ]
+ [ myElement
+ , el [ centerX ] myElement
+ , el [ alignRight ] myElement
+ ]
+
+
+myElement : Element msg
+myElement =
+ el
+ [ Background.color (rgb255 75 192 169)
+ , Font.color (rgb255 255 255 255)
+ , Border.rounded 10
+ , padding 30
+ ]
+ (text "stylish!")
+```
+
+> This is the example in the official `elm-ui` docs:
+https://github.com/mdgriffith/elm-ui
+try the Ellie version: https://ellie-app.com/7vDrXpCckNWa1
+
+In your terminal run:
+
+```
+elm reactor
+```
+
+Open the following page in your web browser:
+http://localhost:8000/src/Main.elm
+
+You should expect to see:
+
+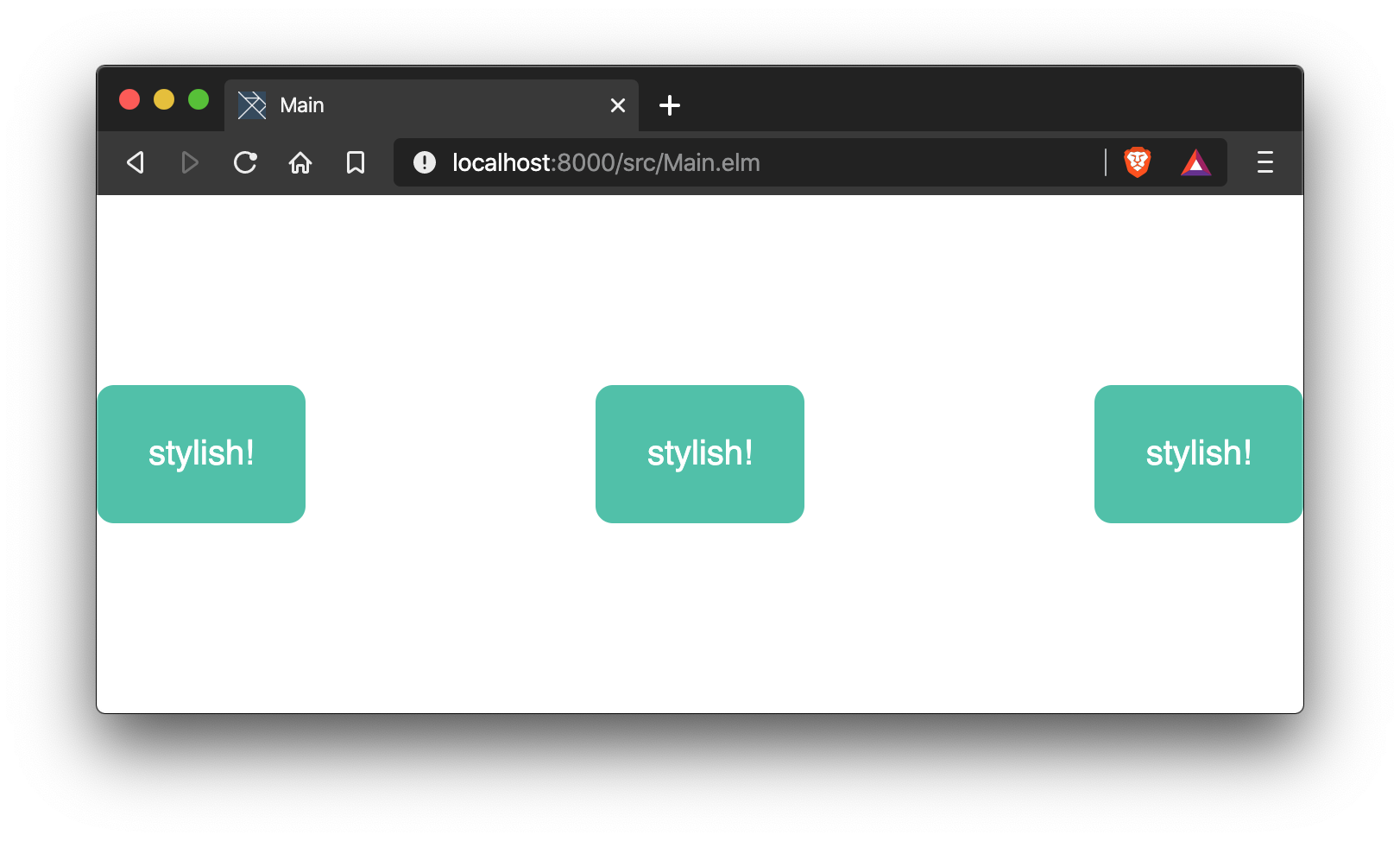
+
+If you open the elements tab of Dev Tools in your browser
+you will see the HTML code (_generated by the `elm` compiler_):
+
+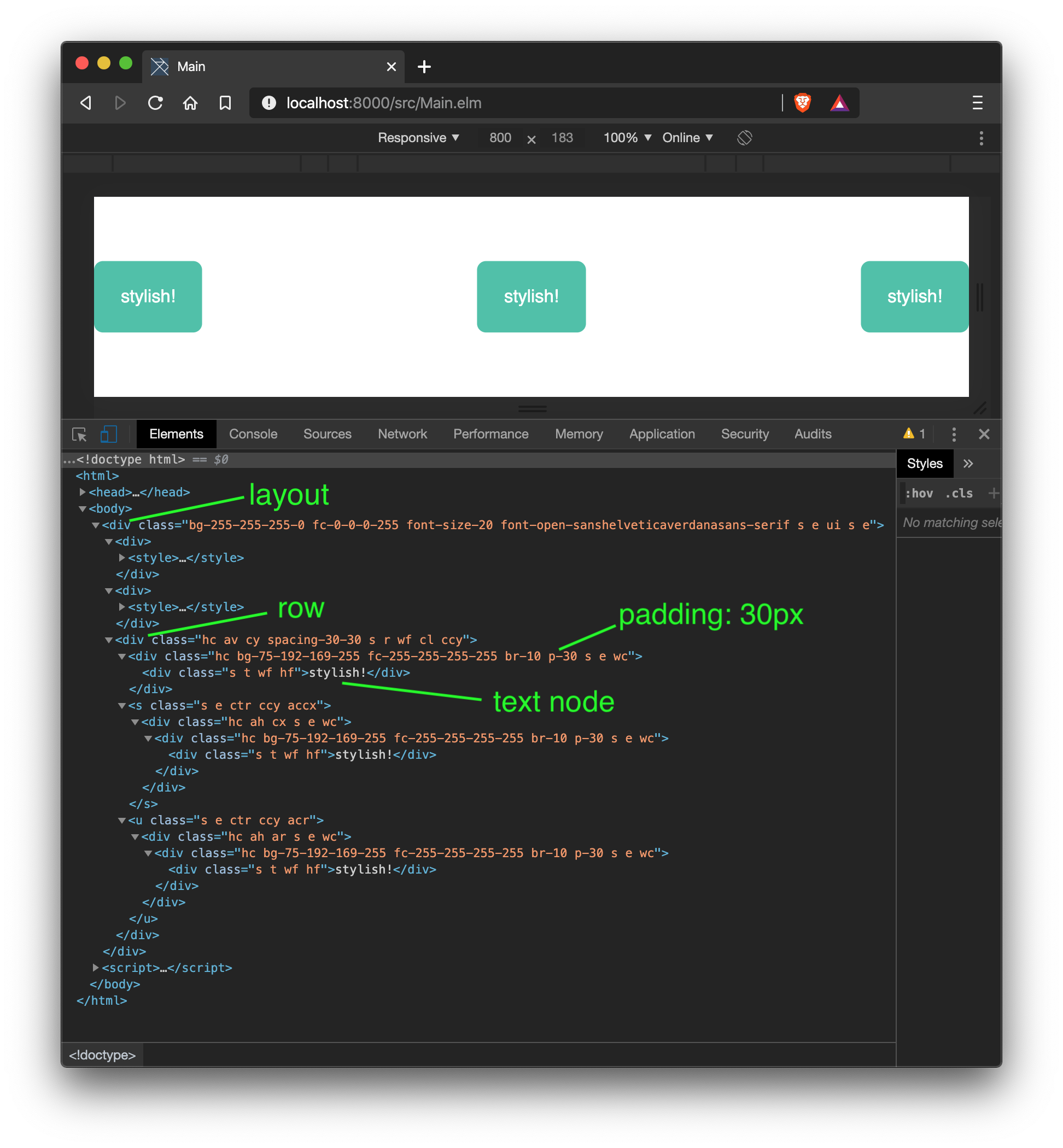
+
+The in-line CSS classes are generated at compile time
+and included in `