-
Notifications
You must be signed in to change notification settings - Fork 531
New issue
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement. We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
Error when slot template renders component with multiple root nodes #111
Comments
And of course, as soon as I type all that up and submit the issue, I start testing out different scenarios and discover an issue with the proposed fix. It get's the Ultimately, it feels like the reliance on the I think the long-term fix may be figuring out how to utilize template Refs to track the elements we want to drag/drop and not go after the underlying |
FWIW, I've created a 2nd example that more accurately reflects what I'm experiencing locally with the 3rd party table: https://codesandbox.io/s/quizzical-cookies-rih8u For these multi-root components, Vue is managing the childNodes list somehow and with the drag/drop Vue is not getting updated on what's has changed so it's still getting some weird behavior. |
I have the same problem with slots and searching for a solution and I've found a PR about this. Is this PR a solution for our problem? #101 |
@jmjurado23 It wasn't a fix for the issue I had as I pulled the fork for that PR and tried it and it didn't work. My understanding is that the usage of slots will automatically introduce the multiroot component. I've got a fork that fixes the issue with Multiroot components. But it also has several other improvements such as moves the repo to using Vite and away from webpack so that we get ESM modules which helps address #117 and a couple of other issues I've come across. I tried to also convert things to using the composition API, but hit a wall on an issue. I've got the current options API version working. I reached out for some help on the Vue Discord but didn't get any clear direction on what the actual difference might be between the two API's that would cause things to work for the Options API but not the Composition API. you can try it out here: https://github.com/jawa-the-hutt/vue.draggable.next there's a bunch of cleanup that's needed on this, so it's not really production ready. I have not touched the Tests to see if they even work. Hence, why I haven't submitted this back up as a PR. |
Do you know why only This is my project https://codesandbox.io/s/github/yaolunmao/el-drag-test I guess it may have something to do with |
I tried to track the code and found that only |
Has this problem not been followed up?Can you expose a hook to customize the specified DOM |
TLDR: reporting a bug and have a proposed fix, but not sure how much of an edge case this is or not.
When inserting a component into the slot, if the component has multiple root nodes in it such that it doesn't get detected by the check at line 26 in renderHelper.js, then the
__draggable_context
property that gets added to the DOM element is added to the wrong node. When this happens, you cannot drag/drop correctly and get a throw error in theonDragStart
event:Cannot read properties of null (reading 'element')
codesandbox.io link
https://codesandbox.io/s/bold-pike-xrddv
Step by step scenario
Take something like this for App.vue
and something like this for the Example.vue component:
The comment line in Example.vue causes the vue compiler to detect multiple root nodes and thus, it surrounds all nodes with an empty text element. So, something like this:
As you can see, this is the
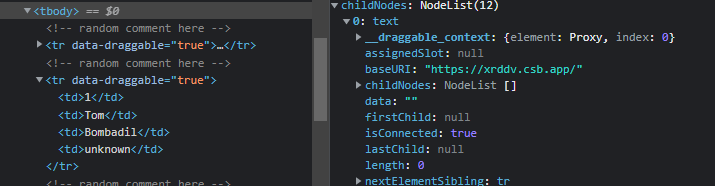
childNodes
of thetbody
element. Here is the firsttext
node expanded and you can see the__draggable_context
property has been added to it.So, when the
onDragStart
event happens and it callsgetContext
here, it fails to find the element correctly and throws the exception.Actual Solution
Here is a proposed solution at codesandbox.io: https://codesandbox.io/s/pedantic-joliot-drjm3.
Please note, for this sandbox, I ended up setting up VueDraggable locally within the sandbox as a subfolder. I do have a PR ready that is the same fix that's contained in this sandbox. You can see the proposed fix in the componentStructure.js file at the very beginning. I'm also manually assigning the
data-draggable
attribute where I need it as that's another symptom of the overall issue and that is that this attribute is not being assigned correctly.What I attempt to do is detect these empty text nodes that Vue 3 is inserting and based on that, I go find the next Sibling and return it instead of the empty text node.
All the above said, I'm not 100% sure this is the best way to go about addressing this scenario. For me specifically, this situation came up with a 3rd party table library where I wanted to be able to drag/sort rows and it wasn't a comment in the
template
section, but it's use of multiple components via renderless functions and jsx elements to render atr
that Vue thinks has multiple root nodes. My use of the comment in thetemplate
section was my attempt to recreate the scenario.Again, not sure I've gone about solving this the "best" way or if there is some other way that would be a better long term fix for this issue.
The text was updated successfully, but these errors were encountered: