diff --git a/README.md b/README.md
index 27470e9..e3baafe 100644
--- a/README.md
+++ b/README.md
@@ -4,7 +4,8 @@ Flexible Assistant Toolkit for GMS2.3+
by Devon Mullane
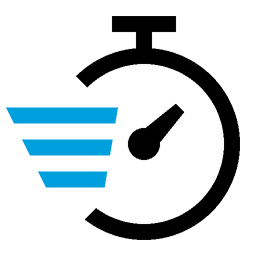
-Check the Wiki for the most up-to-date documentation on each module.
+ Check the Wiki for the most up-to-date documentation on each module.
+ You can also visit the FAST Discord for more interactive help!
FAST is a modular library that provides easy-to-use tools to get GMS developers away from boilerplate tasks and onto building their games. There is no genre-specific code or implementation found here. Instead, FAST focuses powerful, common-use tools that provide general utility to fill the gaps in GML functions and tedious framework tasks. The Core library offers up publisher, event and file frameworks, while modules extend it into more specific tasks such as input handling and resolution scaling, or power-user features such as scripting and databases. In short, FAST can work with every game, and compliment any game made with GMS2.3 or later.
diff --git a/options/ios/options_ios.yy b/options/ios/options_ios.yy
index 1498dc4..ec40469 100644
--- a/options/ios/options_ios.yy
+++ b/options/ios/options_ios.yy
@@ -41,9 +41,7 @@
"option_ios_facebook_app_display_name": "",
"option_ios_push_notifications": false,
"option_ios_apple_sign_in": false,
- "option_ios_podfile_path": "${options_dir}/ios/Podfile",
- "option_ios_podfile_lock_path": "${options_dir}/ios/Podfile.lock",
- "resourceVersion": "1.3",
+ "resourceVersion": "1.0",
"name": "iOS",
"tags": [],
"resourceType": "GMiOSOptions",
diff --git a/options/tvos/options_tvos.yy b/options/tvos/options_tvos.yy
index 5ee2c44..2c3219c 100644
--- a/options/tvos/options_tvos.yy
+++ b/options/tvos/options_tvos.yy
@@ -20,9 +20,7 @@
"option_tvos_display_cursor": false,
"option_tvos_push_notifications": false,
"option_tvos_apple_sign_in": false,
- "option_tvos_podfile_path": "${options_dir}\\tvos\\Podfile",
- "option_tvos_podfile_lock_path": "${options_dir}\\tvos\\Podfile.lock",
- "resourceVersion": "1.3",
+ "resourceVersion": "1.0",
"name": "tvOS",
"tags": [],
"resourceType": "GMtvOSOptions",
diff --git a/options/windows/options_windows.yy b/options/windows/options_windows.yy
index 44cc31c..43cd9af 100644
--- a/options/windows/options_windows.yy
+++ b/options/windows/options_windows.yy
@@ -28,8 +28,7 @@
"option_windows_enable_steam": false,
"option_windows_disable_sandbox": false,
"option_windows_steam_use_alternative_launcher": false,
- "option_windows_use_x64": false,
- "resourceVersion": "1.1",
+ "resourceVersion": "1.0",
"name": "Windows",
"tags": [],
"resourceType": "GMWindowsOptions",
diff --git a/scripts/DsMap/DsMap.gml b/scripts/DsMap/DsMap.gml
index 5bb7907..07443bb 100644
--- a/scripts/DsMap/DsMap.gml
+++ b/scripts/DsMap/DsMap.gml
@@ -1,22 +1,46 @@
/// @func DsMap
/// @wiki Core-Index Data Structures
function DsMap() constructor {
+ /// @param {string} key The key that will be used to retrieve this value.
+ /// @param {mixed} value The value to be assigned to the key.
+ /// @desc Adds the specified key-value pair to the map.
+ /// @returns mixed
static add = function( _key, _value ) {
ds_map_add( pointer, _key, _value );
return _value;
}
+ /// @param {string} key The key that will be used to retrieve this value.
+ /// @param {mixed} value The value to be assigned to the key.
+ /// @desc Adds the specified key-value pair if it doesn't exist, and replaces it if it does.
+ /// @returns mixed
static replace = function( _key, _value ) {
ds_map_replace( pointer, _key, _value );
return _value;
}
+ /// @param key
+ /// @param value to set if key does not have a value already
+ /// @desc If the value read at {key} is undefined, the value at {key} assumes the value of {value}
+ /// @returns mixed
+ static assume = function( _key, _value ) {
+ var _read = get( _key );
+
+ if ( _read == undefined ) {
+ _read = replace( _key, _value );
+
+ }
+ return _read;
+
+ }
+ /// @desc Returns true if the DsMap is empty
static empty = function() {
return ds_map_empty( pointer );
}
+ /// @desc Returns the number of elements in the DsMap
static size = function() {
return ds_map_size( pointer );
diff --git a/scripts/Vec2/Vec2.gml b/scripts/Vec2/Vec2.gml
index 41c4aa0..5b33857 100644
--- a/scripts/Vec2/Vec2.gml
+++ b/scripts/Vec2/Vec2.gml
@@ -14,6 +14,16 @@ function Vec2( _x, _y ) constructor {
y = _y;
}
+ /// @returns real
+ /// @desc Used to get the vectors length.
+ static len = function() {
+ return sqrt( x * x + y * y );
+ }
+ /// @returns real
+ /// @desc Used to get the vectors squared length.
+ static lensqr = function() {
+ return (x * x + y * y);
+ }
/// @param {Vec2} Vec2 The vector to subtract from this one.
/// @returns Vec2
static add = function( _Vec2 ) { return new Vec2( x + _Vec2.x, y + _Vec2.y ); }
@@ -23,13 +33,13 @@ function Vec2( _x, _y ) constructor {
return new Vec2( x - _Vec2.x, y - _Vec2.y );
}
- /// @param {Vec2} Vec2 The vector to multiply this one with.
+ /// @param {Vec2} Vec2 The vector to multiply this one component wise with.
/// @returns Vec2
static multiply = function( _Vec2 ) {
return new Vec2( x * _Vec2.x, y * _Vec2.y );
}
- /// @param {Vec2} Vec2 The vector to divide this one by.
+ /// @param {Vec2} Vec2 The vector to divide this one one component wise by.
/// @returns Vec2
static divide = function( _Vec2 ) {
return new Vec2( x / _Vec2.x, y / _Vec2.y );
@@ -41,6 +51,36 @@ function Vec2( _x, _y ) constructor {
return x * _Vec2.x + y * _Vec2.y;
}
+ /// @param {Vec2} Vec2 The vector to get the cross product with.
+ /// @returns Vec2
+ static cross = function( _Vec2 ) {
+ return (x * _Vec2.x - y * _Vec2.y);
+ }
+ /// @param {Vec2} Vec2 The vector to get the distance to.
+ /// @returns real
+ static dist_to = function( _Vec2 ) {
+ return sqrt((x - _Vec2.x) * (x - _Vec2.x) + (y - _Vec2.y) * (y - _Vec2.y));
+ }
+ /// @param {Vec2} Vec2 The vector to get the squared distance to.
+ /// @returns real
+ static dist_to_sqr = function( _Vec2 ) {
+ return ((x - _Vec2.x) * (x - _Vec2.x) + (y - _Vec2.y) * (y - _Vec2.y));
+ }
+
+ /// @returns Vec2
+ /// @desc Used to normalise the vector to unit length.
+ static normalize = function() {
+ var _len_sqr = lensqr();
+ var _len = 0;
+
+ if ( _len_sqr != 0 ) {
+ _len = sqrt( _len );
+
+ set( x / _len, y / _len);
+ }
+
+ return self;
+ }
/// @desc Returns this vector as an array.
/// @returns array `[ x, y ]`
static toArray = function() {